Observer 1
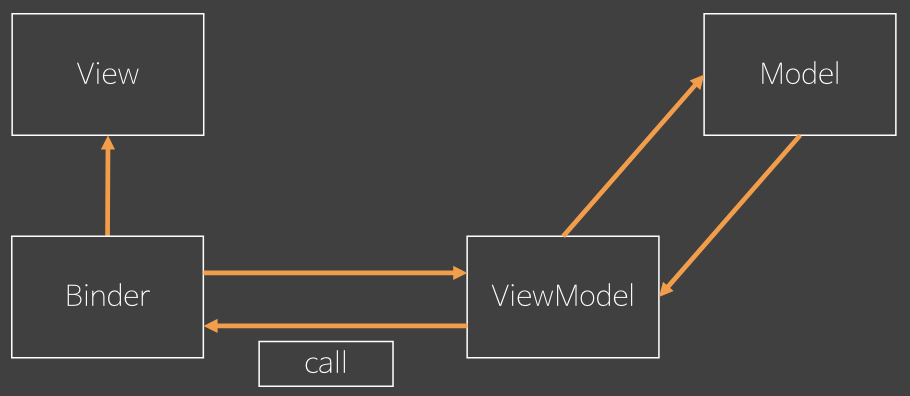
이전의 MVVM 은 observation 을 하고 있지 않음
- Model 의 변화를 자동으로 인식해서 자동으로 Binder 가 움직이지 않았다
- 수동으로 Binder 를 call 했다.
- binder.render(viewmodel);
#
Observer 패턴- Model 변화 → Binder 자동으로 동작
많은 프레임 워크에서는 자동으로 해준다.
Binder 가 ViewModel 을 Observe 한고 렌더링 한다.
Observer(Binder) 가 아닌, ViewModel 이 일을 더 많이해야 한다.
- ViewModel 이 Binder 에게 notify 한다.
- ViewModel 의 변화를 분류해서 보내줘야 한다.
- ViewModel 의 변화를 스스로 알수 있어야 한다.
- defineProperty
- 현시점에서 es5 가 돌아가지 않는 브라우저는 없을 것이다.
- Proxy (es6~, babel 로 converting 불가능)
- 안드로이드 갤럭시 ~s4, 노트2, 윈도우 7(ie9) 는 ES6 미지원
- 신중하게 써야한다
#
Observer 를 형(객체)으로 정의객체의 특정한 메서드 ViewModelListener 를 호출해야 Observing 을 할 수 있다.
- ViewModelListener 가 Type 으로 들어왔을 경우
- viewmodelUpdated 메서드를 호출해서 Observing 을 통보한다.
Observer 패턴은
- 이벤트를 만들어서 보내주는 Subject 가 있고
- 이벤트를 수신하는 Observer 가 있다.
rss 에서는 event emitor 이라고 한다. 끊임없이 이벤트를 만들어 내는것.
뷰모델 확장#
#
필드와 메서드필드
- styles, attributes, properties, events 필드를 가진다.
- isUpdated 뷰 모델의 업데이트 된 항목을 가진다.
- listeners 뷰 모델의 변화를 통보할 리스너들 목록이다.
메서드
- 리스너 추가
- 리스너 삭제
- 리스너에게 isUpdated 를 통보함
- 생성자
- styles, attributes, properties, events 저장